So, I was tasked with a small project to build a nice little script that would target specific tags on a web page and drop Google Analytic Event codes on…
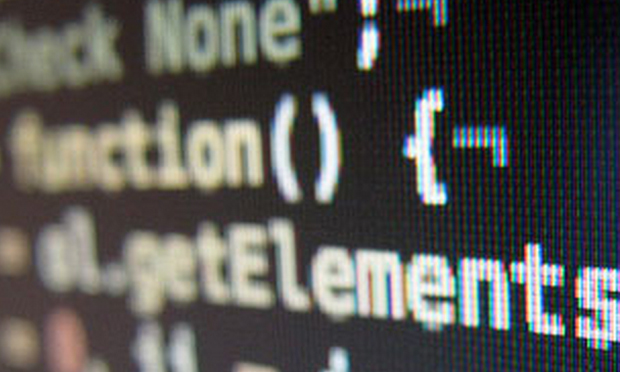
How To: Dynamic Google Analytic Event Tagging Script
So, I was tasked with a small project to build a nice little script that would target specific tags on a web page and drop Google Analytic Event codes on them. The key was to not touch the page code directly. The script is actually lightweight and allows for ease of updating. Here is the code:
/* Dynamic Google Analyics Event Tracker Author: John Steinmetz Version: 1.0 Description: This script takes predefined items on the page and assigned google analytics tracking codes to those items. It allows for dynamic news items to be attached as well. As many tracking events as necessary can be added to the 'events' object without touching the functional code. Considerations: If the page layout or template changes, modifications will be needed to this code to identify the new classes and IDs for tracking. Always use defer="defer" when calling the script to be sure that all page code renders BEFORE the event tagging. */ // Create an array of key value pairs to make solution scalable. You can add as many as you want. var events = [ { element: ".classtotarget1", name: "Example", tag: "Home Page", desc: "Menu Item 1 Click", category: "Navigation" }, { element: ".classtotarget2", name: "Example 2", tag: "Home Page", desc: "Menu Item 2 Click", category: "Navigation" } ] $(document).ready(function() { // Need random strings to serve as data id's for binding elements to our json objects. function guidGenerator() { var S4 = function() { return (((1+Math.random())*0x10000)|0).toString(16).substring(1); }; return (S4()+S4()+S4()); } // initialize variables to be used in the function. // array var news = []; // We can also loop through random items as long as the parent item has a unique ID // loop through all news titles $('.mainnews').each(function(i, obj) { // Give this item a random identifier for use in the tagging process var gui = guidGenerator(); // Add unique class to href to help identify where to put the onclick action on the title $(this).parent().addClass(gui); // dynamically build object var d1 = { element: "."+gui, name: "newsitem", tag: "Home Page", desc: $(this).html(), category: "News" }; // append the object to the array news.push(d1); }); // Combine the static list and the dynamic news list together var combined = events.concat(news); // Loop through all events in the object and append to the HTML $.each(combined, function(index, obj){ var exist = ""; var attr = $(obj.element).attr('onclick'); // If the onclick event already exists, get the data that is there if (typeof attr !== 'undefined' && attr !== false) { // assign that information to a variable that can be appended to the attribute we are creating exist = attr } // Put everything together and create tracking event $(obj.element).attr({ onClick: "_gaq.push(['_trackEvent', '" +obj.tag + "', '" +obj.desc+ "', '" +obj.category+ "']);" + exist }); }); });
You need to have the Google Analytic Async Tracking code on the page for it to work.