One of the most fundamental and confusing things about CSS is targeting elements. Targeting an element means selecting it to apply some styling rule to it. You can also target…
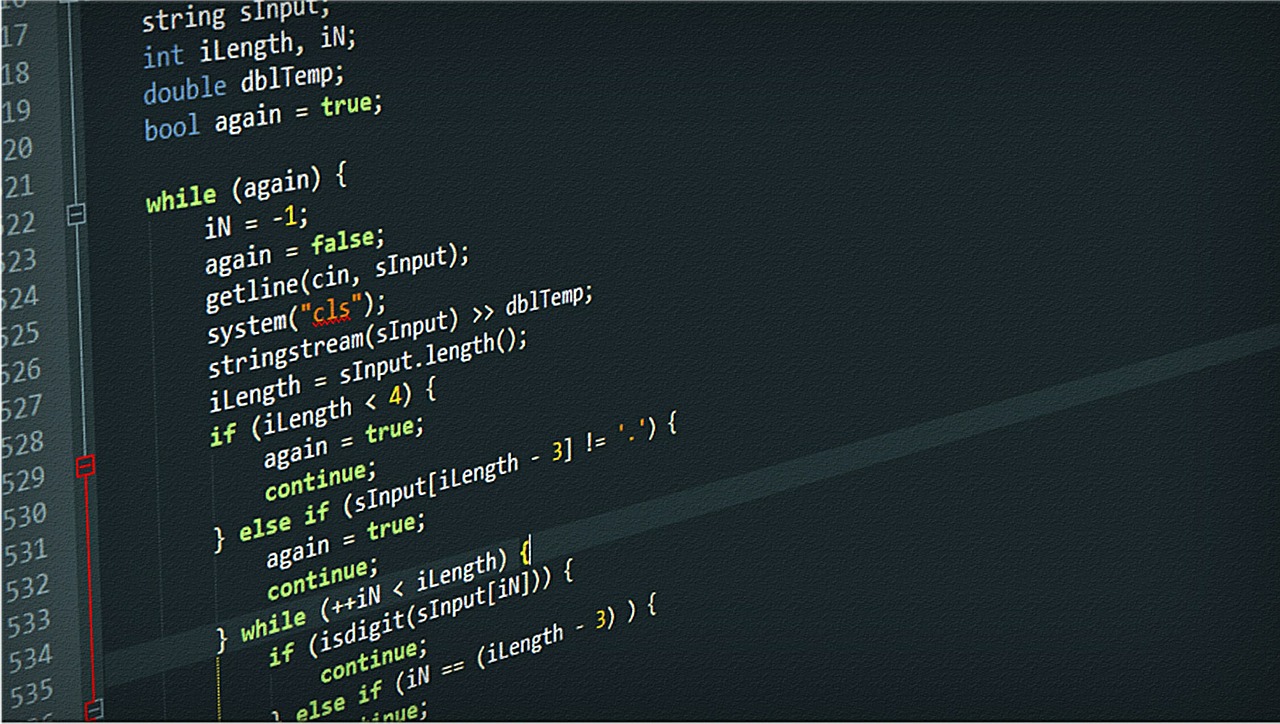
Web Techniques: Targeting Elements in CSS (Beginner)
One of the most fundamental and confusing things about CSS is targeting elements. Targeting an element means selecting it to apply some styling rule to it. You can also target elements for use in JavaScript, but that is another article.
A few of ways to target an item are by element, class, id or other attribute.
First you start with a series of elements. For example’s sake, I’ll add an id and a class to it. Those are the most common targeting applications.
Once you have identified the element you want to apply styling to, you can create CSS rules that “target” that specific element.
/* This will make the font size of the element with attribute id="elephant" 14 pixels */ #elephant { font-size: 14px; } /* This will make the font size of the element with attribute id="tiger" 16 pixels */ #tiger { font-size: 16px; } /* This will make the font color of ALL the elements with attribute class="animal" white */ .animal { color: #ffffff; }
Look at how the items are structured. I used specific animal names as the ids because you should never have more than one id with the same name. Ids in HTML should be unique. Classes, on the other hand are more general and can be applied to multiple elements.
If you look at the rules we applied, both the “elephant” and the “tiger” items will both get styling we assigned to the “animal” class. But, as you can expect, anything we apply to the just the “elephant” id in the css rules, will only apply to that. Same for the “tiger” rules.
It may be necessary sometimes to have multiple classes on an element. You can also target using multiple classes through a method called “chaining”. Take these elements.
As you can see, both elephants and birds are animals so they can share that class. But, even though they are both animals, they still have additional attributes that group them to others.
Now we apply some CSS rules.
/* All elements with the animal class will get the font size of 15 pixels */ .animal { font-size: 15px; } /* All elements with the animal and bird classes will have a bold font */ .animal.bird { font-weight: bold; }
All three items will get a font size of 15px applied. We then applied a “chained” css rule (no spaces) since the div has class=”animal bird” that gave just the items with the second class a bold font.
Note: There is a difference in the css between “.animal.bird” and “.animal .bird”. The first is correct for chaining the way we have it. If you put the space, it means that the HTML applies to a parent/child relationship. See below:
“Cascading” Style Sheets
CSS stands for “Cascading Style Sheets”. This means that if there are multiple stylesheets in an HTML document, they will all combine to form a single stylesheet. The word cascading is used because the application of these styles will start at the first item and cascade down to the last one listed on the page. For example:
Title of Page
This will combine the bootstrap.css with the main.css file to create a single virtual stylesheet. The important thing to remember is that any conflicts in the 2 sheets will be resolved by applying the styles specified in the main.css file.
Specificity
When targeting items with CSS, the more specific the rule, the greater priority of the rule being applied. Take these 2 rules and this block of code.
Because the “.animal.mammal” chained class is more specific than just the “.animal” class, the
will have a font-size of 20px.